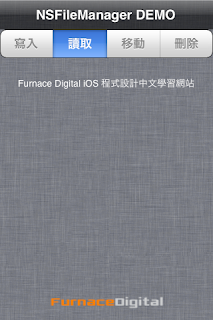
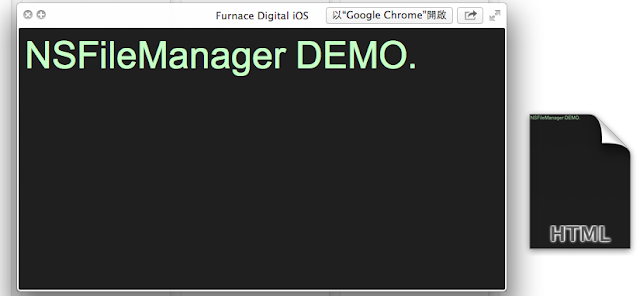
在 iOS SDK 中要對檔案操作免不了要使用到 NSFileManager Class,這個類別提供好用的檔案操作函式,下面就來看看如何使用 NSFileManager 對檔案進行操作。
取得目錄
在開始對檔案操作之前,我們要先知道檔案存放的位置,在 iOS 中提供幾個可供存放檔案的地方,像是 Home、Documents、Library、Tmp 和 Cache 等等資料夾,你可以從以下程式碼獲得取得這些資料夾路徑的方法。
//Home Path
NSString *homePath = NSHomeDirectory();
//Document Path
NSArray *docDirectory = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES);
NSString *documentPath = [docDirectory objectAtIndex:0];
//Library Path
NSArray *libDirectory = NSSearchPathForDirectoriesInDomains(NSLibraryDirectory, NSUserDomainMask, YES);
NSString *libraryPath = [libDirectory objectAtIndex:0];
//Tmp Path
NSString *tmpPath = NSTemporaryDirectory();
//Cache Path
NSArray *cacPath = NSSearchPathForDirectoriesInDomains(NSCachesDirectory, NSUserDomainMask, YES);
NSString *cachePath = [cacPath objectAtIndex:0];
- Documents
這是最常被用來存放檔案的資料夾,也是 Apple 官方建議存放檔案的資料夾,iTunes 備份時會連同此資料夾做備份。
- Library
預設是用來存放程式的相關資訊,像是設定或是執行狀態等等。
- Tmp
暫存用的資料夾,當應用程式結束時,此資料夾也會一並銷燬,不會備份,當系統需要而外資源時,也會優先刪除該資料夾內的東西。
- Cache
位於 Library 中,是 Library 內的子資料夾,此資料夾並不會被 iTunes 備份。
建立新資料夾與檔案
在這一部分,我們會在 Documents 中建立一個新資料夾,並在其中建立空白的檔案等待之後做覆寫,首先是建立新資料夾。
//製作新資料夾的路徑
NSString *newFolderPath = [documentPath stringByAppendingPathComponent:@"新資料夾"];
//建立新資料夾
NSFileManager *fileManager = [NSFileManager defaultManager];
if ([fileManager createDirectoryAtPath:newFolderPath withIntermediateDirectories:YES attributes:nil error:nil])
NSLog(@"新資料夾建立成功");
建立檔案的原理與資料夾相同,唯一的差別是使用 createFileAtPath:contents: attributes: 方法函式來建立檔案。
//製作新檔案的路徑
NSString *newFilePath = [newFolderPath stringByAppendingPathComponent:@"iOS.txt"];
//建立空白新檔案
NSFileManager *fileManager = [NSFileManager defaultManager];
if ([fileManager createFileAtPath:newFilePath contents:nil attributes:nil])
NSLog(@"新檔案建立成功");
對檔案寫入資料
在上述部分我們已經在資料夾中建立了一個空白的檔案,在這部份我們會先取得該檔案的路徑,之後才會進行覆寫的回存的動作。
//寫入內容
NSString *FileContent = @"Furnace Digital iOS 程式設計中文學習網站";
if ([FileContent writeToFile:newFilePath atomically:YES encoding:NSUTF8StringEncoding error:nil])
NSLog(@"檔案覆寫成功");
![]() |
實際檢視所操作的檔案 |
檔案的複製、移動與刪除
在複製、移動與刪除方面,與上述並沒有太大的不同,同樣都是需要先取得檔案的路徑與目標路徑,即可使用下列函式來實作。
//複製
[fileManager copyItemAtPath:@"目前路徑" toPath:@"目標路徑" error:nil]
//移動
[fileManager moveItemAtPath:@"目前路徑" toPath:@"目標路徑" error:nil]
//刪除
[fileManager removeItemAtPath:@"檔案路徑" error:nil]
取得檔案相關屬性
檔案包含許多的相關屬性,像是檔案大小、位置、建立與修改日期、狀態等等,都可以使用 NSDictionary 來取得目標檔案的相關屬性。
NSDictionary *fileAttributes = [fileManager attributesOfItemAtPath:newFilePath error:nil];
for (id key in fileAttributes) {
NSLog(@"key: %@, value: %@ \n", key, [fileAttributes objectForKey:key]);
}
![]() |
iOS.txt 檔案的部份屬性一覽 |
其他輔助判斷
你可以使用下列程式碼,幫助你在實作上判斷檔案使否可以讀寫、執行或是存在。
//判斷檔案是否可讀寫、執行和存在
[fileManager isReadableFileAtPath:@"檔案路徑"]
[fileManager isWritableFileAtPath:@"檔案路徑"]
[fileManager isExecutableFileAtPath:@"檔案路徑"]
[fileManager fileExistsAtPath:@"檔案路徑"]
其他應用
在這部份雖與 NSFileManager 沒什麼關係,但是同樣涉及檔案的操作,下面我們將使用程式碼動態產生一個 index.html 檔案,並將它存放於 Documents 資料夾中。
//建立檔案內容
NSMutableString *myHTML = [[NSMutableString alloc] init];
[myHTML appendFormat:@"<HTML><head><title>Furnace Digital iOS</title></head>"];
[myHTML appendFormat:@"<body bgcolor='#222222'>"];
[myHTML appendFormat:@"<font face='Arial' color='#ccffcc' size='64'>NSFileManager DEMO.</font>"];
[myHTML appendFormat:@"</body>"];
//取得 Document Path
NSArray *docDirectory = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES);
NSString *documentPath = [docDirectory objectAtIndex:0];
//取得檔案路徑
NSString *newFilePath = [documentPath stringByAppendingPathComponent:@"index.html"];
//寫入
[myHTML writeToFile:newFilePath atomically:YES encoding:NSUTF8StringEncoding error:nil];
![]() |
index.html 的執行結果 |
Thank you
回覆刪除You’re welcome.
刪除